7. Hashing - I
In this lab we continue implementing our own data structure library.
You will implement the following UML in Java:
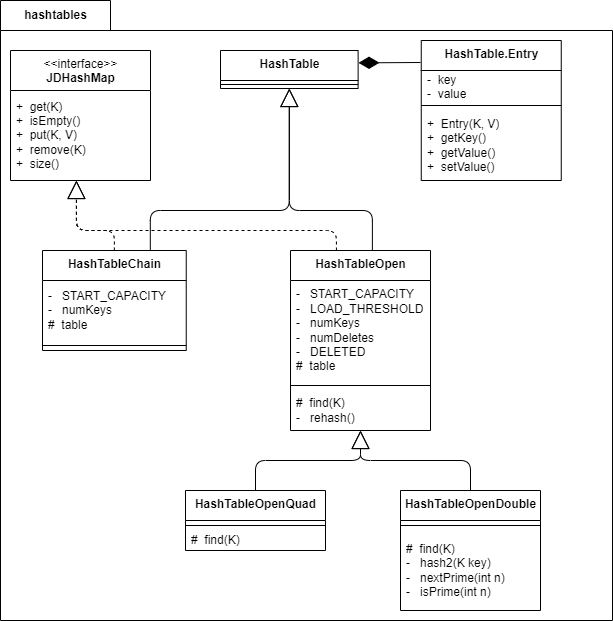
7.1. Hashing - Start
Create a package
hashtables
.Create the interface
JDHashMap
.
7.2. Hashing - Hashtable
Create the structure of the generic class
HashTable
.Create the generic and static class
Entry<K, V>
insideHashTable
.It contains only two data fields.
Implement the constructor
Entry(K key, V value)
.Implement the methods
K getKey()
,V getValue()
andV setValue(V val)
Implement the method
toString()
.
7.3. Hahsing - HashTableChain
Create the generic class
HashTableChain
.You will implement the constructor
HashTableChain()
It contains only three data fields:
ArrayList<Entry<K, V>>[] table
static final int START_CAPACITY
int numKeys
Implement all the function from the interface.
Make sure that your code works.
ENSURE WE HAVE RECORDED YOUR COMPLETION. FAILURE TO DO SO WILL RESULT IN A GRADE OF 0!