3. BST
In this lab you will start implementing your own data structure library.
You will implement the following UML in Java:
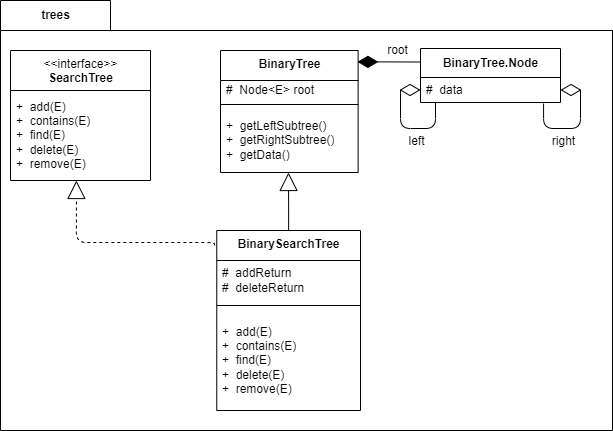
3.1. BST - Start
Create a package
trees
.Create the interface
SearchTree
.
3.2. BST - BinaryTree
Create the structure of the generic class
BinaryTree
.It should implement
Serializable
.
Create the generic and static class
Node
.It should implement
Serializable
.It contains only three data fields.
Implement the constructor
Node(E data)
.Implement the method
toString()
.
Now finish the class
BinaryTree
.You will implement three constructors:
BinaryTree()
BinaryTree(Node<E> root)
BinaryTree(E data, BinaryTree<E> leftTree, BinaryTree<E> rightTree)
3.3. BST - BinaryTreeSearch
Create the generic class
BinarySearchTree
.The generic type
E
should inherit fromComparable
.The class should inherit from
BinaryTree
.And it should implement
SearchTree
Implement all the function from the interface.
Make sure that your code works.
3.4. LeetCode
If you are done, you can start doing the following problems on LeetCode: