6. Red-Black Trees
In this lab we continue implementing our own data structure library.
You will implement the following UML in Java:
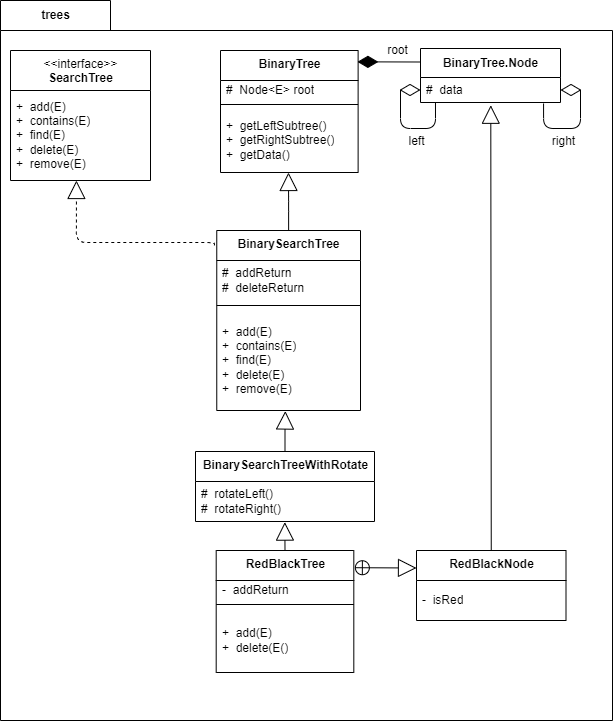
6.1. Red-Black Trees - Start
Finish the class
BinarySearchTreeWithRotate
witht the following methods:rotateRight(E)
rotateLeft(E)
1package trees;
2
3/**
4 * This class extends the BinarySearchTree by adding the rotate operations.
5 * Rotation will change the balance of a search tree while preserving the search tree property.
6 * Used as a common base class for self‐balancing trees.
7 * @param <E> type of the data contained in the tree
8 */
9public class BinarySearchTreeWithRotate<E extends Comparable<E>> extends BinarySearchTree<E>{
10
11 /**
12 * Method to perform a right rotation.
13 * @post root.right is the root of a binary search tree,
14 * root.right.right is raised one level,
15 * root.right.left does not change levels,
16 * root.left is lowered one level,
17 * the new root is returned.
18 * @param root is the root of a binary search tree
19 * @return The new root of the rotated tree
20 */
21 protected Node<E> rotateRight(Node<E> root) {
22 }
23
24 /**
25 * Method to perform a left rotation.
26 * @post root.left is the root of a binary search tree,
27 * root.left.left is raised one level,
28 * root.left.right does not change levels,
29 * root.right is lowered one level,
30 * the new root is returned.
31 * @param root is the root of a binary search tree
32 * @return The new root of the rotated tree
33 */
34 protected Node<E> rotateLeft(Node<E> root) {
35 }
36
37}
6.2. Red-Black Trees - class
Create the structure of the generic class
RedBlackTree
.It should inherit from
BinarySearchTreeWithRotate
.
1package trees;
2
3/**
4 * Self-balancing binary search tree using algorithm defined by
5 * Leo Guibas and Robert Sedgewick.
6 * @param <E> Type contained in the tree.
7 */
8public class RedBlackTree<E extends Comparable<E>> extends BinarySearchTreeWithRotate<E> {
9}
Create the generic and static class
RedBlackNode
.It should inherit from
Node
.It contains only three constant data field.
Implement the constructor.
1 /**
2 * Class to represent a Red-Black node
3 * @param <E> The data type of items stored in the node. Must be Comparable
4 */
5 private static class RedBlackNode<E> extends Node<E> {
6 /**
7 * Constructor to create a node with the default color of red with the given data
8 * @param data The data item to be stored in the node
9 */
10 public RedBlackNode(E data) {
11 }
12
13 //Methods
14 /**
15 * Return a String representation of the node
16 * @return A String representation of the node
17 */
18 @Override
19 public String toString(){
20 }
21
22 }
Now finish the class
RedBlackTree
.
1package trees;
2
3/**
4 * Self-balancing binary search tree using algorithm defined by
5 * Leo Guibas and Robert Sedgewick.
6 * @param <E> Type contained in the tree.
7 */
8public class RedBlackTree<E extends Comparable<E>> extends BinarySearchTreeWithRotate<E> {
9 /**
10 * Add start method
11 * @param item The new element.
12 * @return true if the object is inserted;
13 * false if the object already exists in the tree.
14 */
15 @Override
16 public boolean add(E item){
17 }
18
19 /**
20 * Recursive add method
21 * @param localRoot The local root of the Red Black Tree
22 * @param item The item to be inserted
23 * @return The new local root
24 */
25 private Node<E> add(RedBlackNode<E> localRoot, E item){
26 }
27
28 /**
29 * Check to see whether both children of the given node are red.
30 * If so, make them black and change the localRoot's color to red.
31 * @param localRoot The node to check whether both children are red or not
32 */
33 private void moveBlackDown(RedBlackNode<E> localRoot){
34 }
35
36}
6.3. Red-Black Trees - Test
You can use the following function to test your code:
1public static void rbt_tree(){
2 RedBlackTree<String> testOne = new RedBlackTree<String>();
3 testOne.add("The");
4 testOne.add("quick");
5 testOne.add("brown");
6 testOne.add("fox");
7 testOne.add("apple");
8 testOne.add("cat");
9 testOne.add("hat");
10 System.out.println(testOne.toString());
11
12 RedBlackTree<Integer> testTwo = new RedBlackTree<Integer>();
13 testTwo.add(30);
14 testTwo.add(40);
15 testTwo.add(15);
16 testTwo.add(25);
17 testTwo.add(90);
18 testTwo.add(80);
19 testTwo.add(70);
20 testTwo.add(85);
21 testTwo.add(15);
22 testTwo.add(72);
23 System.out.println(testTwo.toString());
24
25 RedBlackTree<String> testThree = new RedBlackTree<String>();
26 testThree.add("Now");
27 testThree.add("is");
28 testThree.add("time");
29 testThree.add("for");
30 testThree.add("all");
31 testThree.add("good");
32 testThree.add("men");
33 testThree.add("to");
34 testThree.add("come");
35 testThree.add("to");
36 testThree.add("the");
37 testThree.add("aid");
38 testThree.add("of");
39 testThree.add("the");
40 testThree.add("party");
41 System.out.println(testThree.toString());
ENSURE WE HAVE RECORDED YOUR COMPLETION. FAILURE TO DO SO WILL RESULT IN A GRADE OF 0!