4. Heaps
You will implement the following UML in Java:
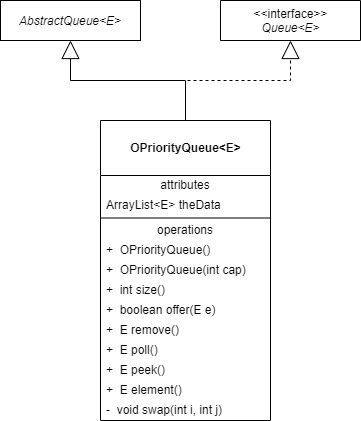
4.1. Priority Queue
Create the generic class
OPriorityQueue
.The generic type should extends
Comparable
.OPriorityQueue
extends the Java API classAbstractQueue
.It also implements the Java API interface
Queue
.
Implement the attribute and the methods of the class:
boolean offer(E item)
: Inserts an item into the queue. Returnstrue
if successful; returnfalse
if the item could not be inserted.E remove(E item)
: Removes the smallest entry and returns it if the queue is not empty. If the queue is empty, throws aNoSuchElementException
.E poll(E item)
: Removes the smallest entry and returns it if the queue is not empty. If the queue is empty, returns anull
.E peek(E item)
: Returns the smallest entry without removing it. If the queue is empty, returns anull
.E element(E item)
: Returns the smallest entry without removing it. If the queue is empty, throws aNoSuchElementException
.
4.2. Breadth-First Traversal
Implement the following algorithm:
void breadth_first_search(OPriorityQueue queue, BinarySearchTree bst){
Insert root node in queue;
while(queue not empty){
node = remove node from queue;
visit(node);
insert node.left in queue;
insert node.right in queue;
}
Test it on several binary trees.
ENSURE WE HAVE RECORDED YOUR COMPLETION. FAILURE TO DO SO WILL RESULT IN A GRADE OF 0!